Simple Java Mail Modules
Simple Java Mail core features can be used without any external libraries, but you can add some features by depending on additional modules, which bring in some additional dependencies of their own.
The Core Module is included by default when you add Simple Java Mail. The other modules from the implementation layer can be added to complement Simple Java Mail.
On the other hand, if you depend on one of the integration modules, you get Simple Java Mail automatically as well. Again you can add the other modules from the implementation layer to complement Simple Java Mail.
The core dependencies are:
- org.slf4j:slf4j-api
- com.sun.mail:jakarta.mail
- com.sun.activation:jakarta.activation
- jakarta.xml.bind:jakarta.xml.bind-api
- jakarta.annotation:jakarta.annotation-api
- com.sanctionco.jmail:jmail (address validation)
- com.pivovarit:throwing-function (improved Streams api)
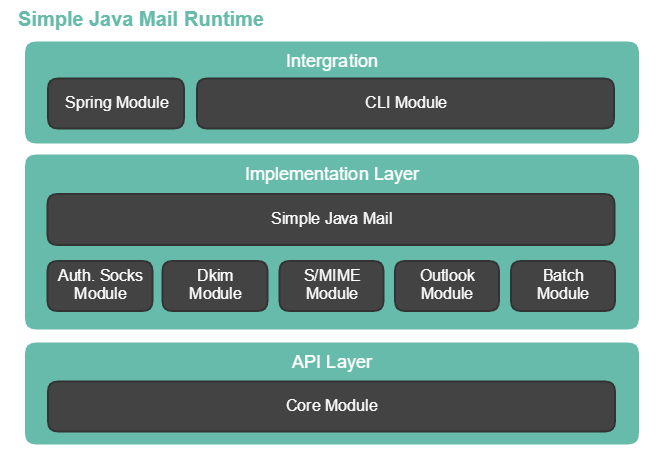
Batch and Clustering support
To enable support for async / batch mode sending using connection pooling, and clustering (see Configuration), include the following library:
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>batch-module</artifactId>
</dependency>
This adds the following 3rd party dependencies:
This allows you to send emails asynchronous with configuration options for thread management and SMTP connection pooling. See configuration for more details.
Note that with the batch-module enabled, the JVM won't shut down by itself anymore, as the connection pool stays alive until
shutdown manually. To do this, just call mailer.shutdownConnectionPool()
(repeat with each mailer you might have
in a cluster).
Authenticated (SOCKS) proxy support
To enable support for authenticated SOCKS proxy servers (see Features), include the following library:
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>authenticated-socks-module</artifactId>
</dependency>
This adds no additional 3rd party dependencies.
Outlook support
To enable support for parsing Outlook messages (see Features), include the following library:
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>outlook-module</artifactId>
</dependency>
This adds the following 3rd party dependencies:
- com.esotericsoftware:kryo
- de.javakaffee:kryo-serializers
- org.simplejavamail:outlook-message-parser
- Of which that last in turn adds:
- org.apache.poi:poi
- org.apache.poi:poi-scratchpad
DKIM support
To enable support for DKIM signing (see Features), include the following library:
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>dkim-module</artifactId>
</dependency>
This adds the following 3rd party dependencies:
S/MIME support
To enable support for sending and reading S/MIME signed / encrypted email, (see Features), include the following library:
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>smime-module</artifactId>
</dependency>
This adds the following 3rd party dependencies:
CLI support
Normally, you would not need the CLI module on the class path, unless you wish to invoke the
static void main
method from Java (see also how to use CLI).
To do so, include the following module:
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>cli-module</artifactId>
</dependency>
This adds the following 3rd party dependencies:
Note: Simple Java Mail core has a 'provided' dependency on com.github.therapi:therapi-runtime-javadoc-scribe which burns Javadoc into Simple Java Mail at compile time. This cannot be made optional. If this is a real concern, an option could be to provide two versions of Simple Java Mail: one with embedded Javadoc and one without (incompatible with the Cli Module). This is currently not in scope.
Spring support
To enable Spring support (see Configuration), include the following module:
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>spring-module</artifactId>
</dependency>
This adds the Spring core dependency, which is assumed to be on the classpath already.
Apache Karaf support
To enable Apache Karaf support, include the following module:
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>karaf-module</artifactId>
</dependency>
NOTE: the artifact is currently temporarily named: 'simplejavamail-karaf-feature'. It will be named correctly in the next release.